Java is a widely used programming language, and understanding exceptions is crucial for developing robust applications. Exceptions represent errors that occur during the execution of a program, and Java has a well-defined mechanism for handling them. The language classifies exceptions into two types: checked exceptions and unchecked exceptions. Knowing the distinction between these two is essential for writing reliable and maintainable code, as they govern how your code will respond to errors.
This article will delve deep into the differences between checked and unchecked exceptions, when to use them, and how they align with best practices in Java development. We will also explore how understanding exceptions can improve your career prospects by attending a quality Java training institute, like the ones offering Java J2EE training in Chennai.
What Are Exceptions in Java?
Before diving into the differences, it’s important to understand what exceptions are. In Java, an exception is an event that disrupts the normal flow of a program’s execution. When an error occurs, Java generates an exception, and the program terminates abnormally unless the exception is handled properly.
Java provides a powerful mechanism to handle exceptions via the try, catch, finally, and throw keywords. This allows developers to manage errors gracefully without crashing the application.
Checked Exceptions
Checked exceptions are the exceptions that are checked at compile time. This means that when a method is defined to throw a checked exception, the caller of that method must handle the exception using a try-catch block or propagate it further using the throws keyword.
These exceptions derive from the java.lang.Exception class, except for RuntimeException and its subclasses.
Characteristics of Checked Exceptions:
- Compile-Time Checking: The compiler ensures that checked exceptions are either caught or declared in the method signature.
- Must Be Handled: If a method throws a checked exception, it must be handled explicitly, either by a try-catch block or by declaring the exception in the method signature using the throws clause.
- Predominantly External Errors: Checked exceptions are generally related to external resources, such as files, databases, or network connections.
Example of Checked Exceptions:
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class CheckedExceptionExample {
public static void main(String[] args) {
try {
File file = new File(“test.txt”);
FileReader fr = new FileReader(file);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, the FileReader constructor throws an IOException, a checked exception. Java forces the developer to handle this exception to ensure the application doesn’t crash when the file isn’t found or readable.
When to Use Checked Exceptions:
- IO Operations: Checked exceptions are common in Input/Output operations. For instance, dealing with file reading and writing operations, database connectivity, and network operations often involves checked exceptions.
- Business Logic Errors: These are appropriate when you expect certain scenarios that can disrupt the flow, like invalid user input or unavailability of a resource, but they don’t imply a bug in the code.
By learning how to handle such situations effectively, your skills will improve significantly, especially when you complete your Java J2EE training programs that focus on robust Java development.
Unchecked Exceptions:
Unchecked exceptions, on the other hand, are exceptions that are not checked at compile time. These exceptions are derived from the RuntimeException class. They occur due to programming errors, such as logic issues or improper use of APIs, which generally happen during runtime.
Unlike checked exceptions, unchecked exceptions do not need to be explicitly handled, meaning the compiler does not enforce handling them.
Characteristics of Unchecked Exceptions:
- Runtime Occurrence: Unchecked exceptions occur during the program’s execution, typically due to programming errors like arithmetic mistakes or null references.
- No Handling Obligation: You are not required to handle unchecked exceptions using try-catch blocks, although you can if you choose to.
- Typically Logic Errors: These exceptions generally occur due to logical errors in the code, such as ArrayIndexOutOfBoundsException or NullPointerException.
Example of Unchecked Exceptions:
public class UncheckedExceptionExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[5]); // ArrayIndexOutOfBoundsException
}
}
In this example, accessing an invalid index in an array throws an ArrayIndexOutOfBoundsException, which is an unchecked exception. Since this error is due to a logical flaw (accessing an index that doesn’t exist), Java does not force you to handle it at compile time.
When to Use Unchecked Exceptions:
- Programming Errors: Unchecked exceptions are ideal when dealing with programming errors such as invalid arguments, null values, or arithmetic errors. For example, a NullPointerException occurs when you try to call a method on a null object reference.
- API Design: When designing APIs, unchecked exceptions can be thrown in case of misuse of the API methods. If the client code calls a method with an illegal argument, an IllegalArgumentException (an unchecked exception) may be thrown.
Understanding these concepts will not only help you become a better Java developer but also improve your job prospects, especially when you join a Java training with placement in Chennai. A comprehensive understanding of exceptions is a core topic in any advanced Java training course.
Key Differences Between Checked and Unchecked Exceptions
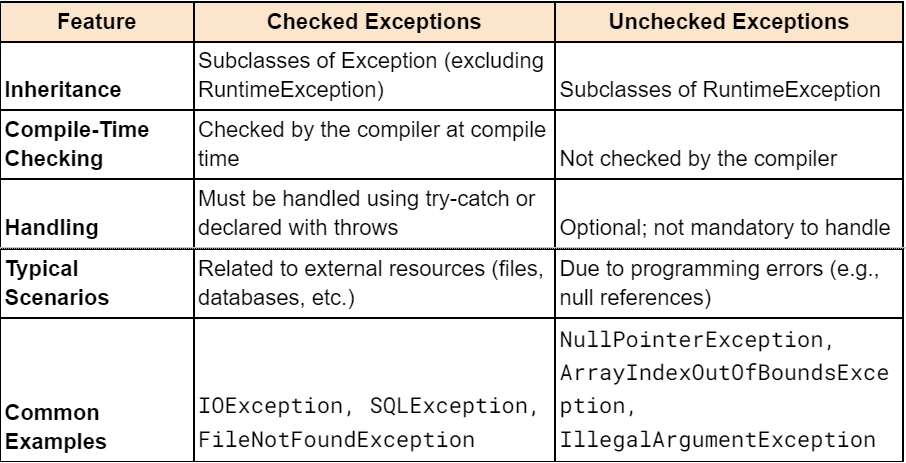
Best Practices for Exception Handling
- Use Checked Exceptions for Recoverable Errors: Checked exceptions are suited for recoverable conditions where the program can do something meaningful in response. For example, if a file is not found, you can prompt the user to provide another file.
- Use Unchecked Exceptions for Programming Errors: Unchecked exceptions are preferable for programming logic errors that can be avoided by writing better code. These errors, such as null references or index out of bounds, typically indicate bugs.
- Avoid Overusing Checked Exceptions: While checked exceptions ensure the handling of specific errors, overuse can make the code clunky and difficult to read. Use checked exceptions when necessary but avoid excessive exception declarations.
- Document the Exceptions: When designing APIs or writing methods that throw exceptions, document them clearly in the method signature. This helps developers understand what exceptions to expect and how to handle them.
- Differences in Usage Knowing when and how to use checked and unchecked exceptions is essential for developing reliable Java applications. Let’s break down the scenarios where each type of exception is most appropriate:
Checked Exceptions: For Foreseeable and Recoverable Errors
Checked exceptions are intended to handle foreseeable conditions that could occur during the execution of a program. These are situations where recovery is expected, and the programmer should handle the exception appropriately. For instance, if you’re working with file handling, network operations, or database access, using checked exceptions is a good practice because these operations often involve external resources and are prone to failures. When an error occurs in such cases, the application can handle the issue gracefully instead of crashing.
Here are some typical use cases for checked exceptions:
- File Not Found: If a file is not available at the specified location, a FileNotFoundException will be thrown. This is a recoverable situation, as the program can prompt the user to select a different file or notify them that the file doesn’t exist.
- SQL Errors: When working with databases, if a query execution fails, it throws a SQLException. In this case, the program can attempt to execute an alternative query or notify the user about the issue.
- Network Timeouts: When connecting to a network resource, a timeout might occur, triggering an IOException. The program can either retry the operation or alert the user.
Using checked exceptions ensures that such critical scenarios are managed properly and that developers are forced to handle them at compile time, ensuring that necessary steps are taken to avoid runtime failures.
Unchecked Exceptions: For Programming Errors
Unchecked exceptions, on the other hand, are generally used to handle programming errors. These errors are often bugs in the code that developers should fix rather than try to recover from. For example, if a program attempts to divide by zero or access a null object reference, these situations are not meant to be “recovered” from but rather indicate errors in logic that need to be corrected.
Typical use cases for unchecked exceptions include:
- Null Pointer Errors: A NullPointerException occurs when the code tries to call a method or access a property on a null object. This is a sign of a bug in the program, and there’s usually no way to recover without fixing the underlying issue.
- Array Index Errors: If an attempt is made to access an array index that doesn’t exist, an ArrayIndexOutOfBoundsException is thrown. This again indicates a coding mistake rather than an exceptional condition that the program can recover from.
- Illegal Argument: An IllegalArgumentException is thrown when a method receives an argument that it doesn’t expect. For instance, passing a negative number to a method that calculates factorials would result in this type of exception.
In these scenarios, the errors are best addressed by fixing the code rather than trying to handle the exception and continue executing the program. By using unchecked exceptions, developers can write cleaner code, since there’s no need to force error handling in situations where recovery is either impossible or doesn’t make sense.
Best Practices for Using Exceptions in Java
Understanding the differences between checked and unchecked exceptions is only part of the equation. To be a proficient Java developer, you must also adhere to best practices when working with exceptions. Here are some recommendations:
- Use Checked Exceptions for Recoverable Conditions: For cases where the application can recover (e.g., file or network errors), use checked exceptions. These errors should be handled explicitly, ensuring the user is notified and provided with alternative options where applicable.
- Reserve Unchecked Exceptions for Programming Errors: Unchecked exceptions should be used for conditions that are indicative of bugs or logical mistakes in the code. These errors should be identified and fixed during the development phase.
- Avoid Overusing Checked Exceptions: While checked exceptions have their place, overusing them can clutter your code with unnecessary try-catch blocks. For example, if you have an operation that’s unlikely to fail, consider using unchecked exceptions to keep your code clean and maintainable.
- Document Exceptions Clearly: Always document which exceptions your methods throw, especially when using checked exceptions. This helps other developers understand the types of errors they need to handle and how to best approach them.
- Consider Custom Exceptions: If your application involves specific business logic that doesn’t map cleanly to existing Java exceptions, consider creating your own custom exceptions. These should extend either Exception (for checked) or RuntimeException (for unchecked) depending on your use case.
How Understanding Exceptions Can Boost Your Career
Mastering exception handling in Java can significantly impact your career as a developer. Not only does it allow you to write more robust and reliable applications, but it also makes your code easier to maintain and debug. This knowledge is especially crucial in environments where Java is used for large-scale applications, such as in enterprise software development or financial services.
Attending a Java training that covers exception handling in depth can help you refine these skills. Comprehensive training courses like Java training with placement not only teach the fundamentals of exception handling but also offer hands-on projects that simulate real-world situations, preparing you for challenges in your future career.
Conclusion
In summary, the distinction between checked and unchecked exceptions in Java is crucial for understanding how to handle errors effectively. Checked exceptions are best used for predictable, recoverable situations, while unchecked exceptions are reserved for programming errors that need fixing. By mastering this distinction and applying best practices, you can write cleaner, more robust Java applications.
Moreover, if you’re looking to further enhance your understanding of Java, enrolling in a Java training institute in Chennai can offer the structured learning environment needed to master these skills.